Understanding Trees, a Fundamental Data Structure
- compnomics
- Mar 4, 2024
- 2 min read
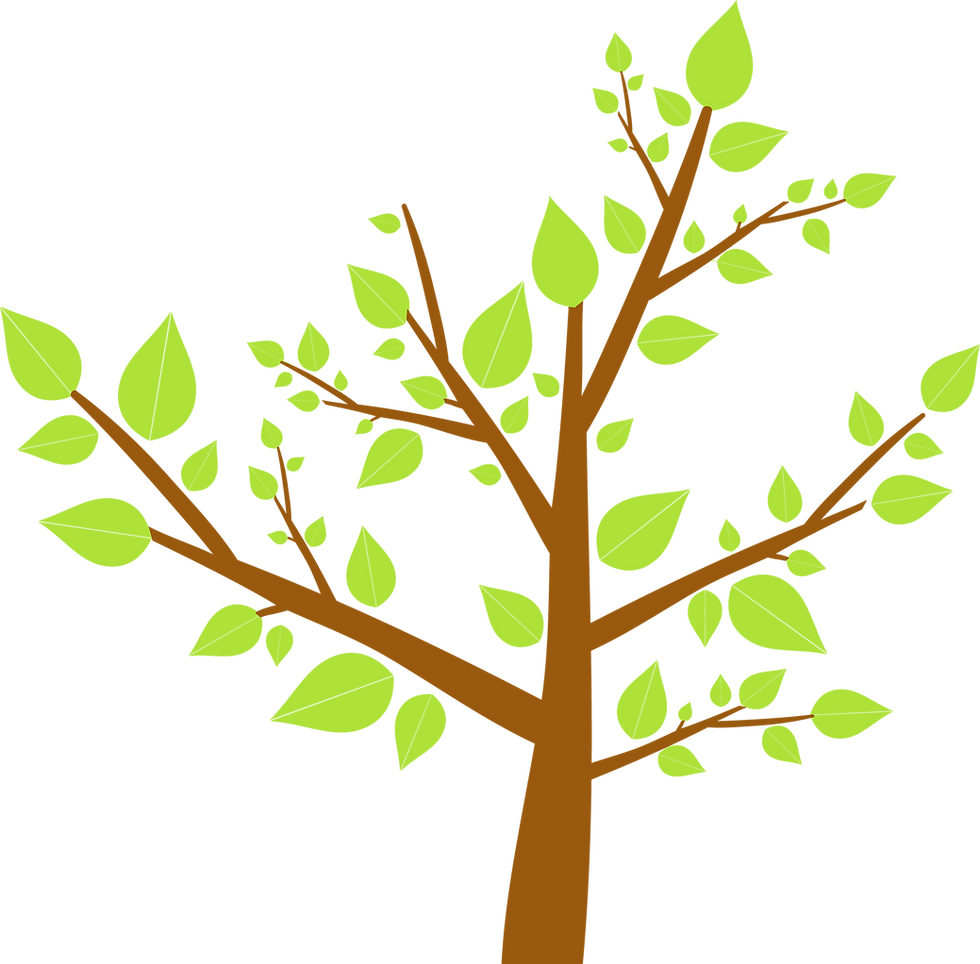
In the realm of data structures, trees hold a special place. Unlike flat lists or arrays, trees offer a hierarchical structure, resembling an actual tree with branches and leaves. This blog post delves into the definition and basic concepts of trees, equipping you with a solid foundation for exploring their applications in computer science.
What is a Tree?
Imagine an organizational chart of a company, with a CEO at the top and departments branching out below. This hierarchical structure is a perfect analogy for a tree data structure.
Nodes: The fundamental building blocks of trees are nodes, which contain data and references to other nodes.
Root Node: The topmost node in the tree is called the root node. It has no parent node.
Child Nodes: Nodes directly connected to a parent node are called child nodes.
Parent Node: A node that has one or more child nodes is called a parent node.
Leaf Node: Nodes without any child nodes are called leaf nodes.
Basic Concepts:
Tree Terminology: Familiarize yourself with terms like subtree (a portion of the tree rooted at a specific node), degree (the number of child nodes a node has), and binary tree (a special type of tree where each node can have at most two child nodes).
Recursive Nature: Trees are inherently recursive, meaning a tree can be defined as a collection of nodes where each node can be a tree itself (except for leaf nodes). This recursive definition allows for efficient representation of hierarchical relationships.
Common Operations on Trees:
Traversal: Visiting each node in the tree systematically. Common traversal methods include in-order, pre-order, and post-order, each following a specific order for visiting nodes and their children.
Searching: Finding a specific node with a particular value within the tree. The hierarchical structure allows for efficient searching algorithms compared to linear searches in flat data structures.
Insertion: Adding a new node to the tree while maintaining the hierarchical relationships and order.
Deletion: Removing a node from the tree while ensuring the structure remains valid.
Applications of Trees:
Trees have a wide range of applications in computer science, including:
File Systems: Hierarchical organization of directories and files on a computer.
Search Algorithms: Binary search trees provide efficient searching for sorted data.
Decision Trees: Used in machine learning to classify data based on a series of questions.
Symbol Tables: Storing and retrieving symbols and their meanings in compilers and interpreters.
Syntax Analysis: Parsing the structure of code or expressions in programming languages.
The Power of Hierarchy:
By understanding trees, you unlock a powerful tool for organizing and manipulating data in a hierarchical fashion. This knowledge empowers you to tackle problems in various domains, from file systems to machine learning algorithms. So, next time you encounter a tree data structure, remember its branching structure and the efficient way it organizes information.
コメント